[무료] 스프링부트 시큐리티 & JWT 강의 - 인프런 | 강의
스프링부트 시큐리티에 대한 개념이 잡힙니다., - 강의 소개 | 인프런...
www.inflearn.com
0. 전반적 흐름
Spring Security는 로그인 url로 (ex. /login) 로그인 요청이 들어오면 이를 낚아채서 자신이 로그인을 진행시킨다.
이때 로그인이 성공적으로 완료되면 Security 자신만의 세션에 유저 정보를 저장하는데, 이 세션이 바로 ContextHolder이다.
ContextHolder에 저장될 수 있는 객체의 타입은 Authentication 타입으로 제한된다.
이 Authentication 객체 안에는 유저 정보가 객체 타입으로 저장되는데, 이 유저 정보 객체도 타입이 UserDetails로 제한된다.
결론적으로 ContextHolder안에 저장된 Authentication 객체 안에 저장된 UserDetails 타입의 객체에 유저 정보가 저장되는 것이다. 따라서 우리는 UserDetails를 implements하는 다양한 PrincipalDetails를 만들어 유저 정보를 관리하면 된다.
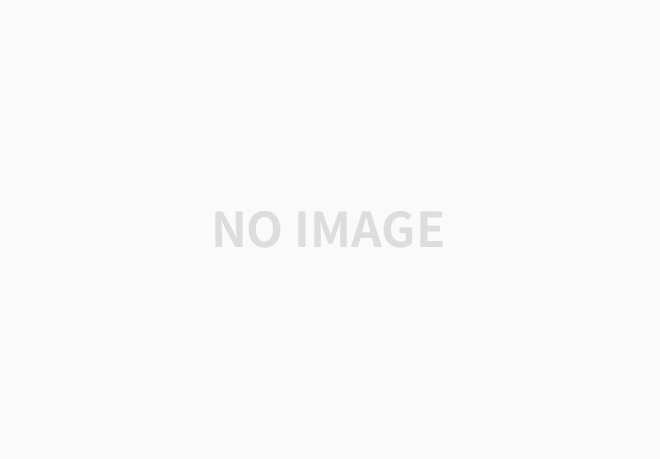
1. PrincipalDetails 뜯어보기
package com.cos.security1.config.auth;
import com.cos.security1.model.User;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import java.util.ArrayList;
import java.util.Collection;
public class PrincipalDetails implements UserDetails {
private User user;
public PrincipalDetails(User user) {
this.user = user;
}
// 해당 유저의 권한을 리턴
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
Collection<GrantedAuthority> collection = new ArrayList<>();
collection.add(new GrantedAuthority() {
@Override
public String getAuthority() {
return user.getRole();
}
});
return collection;
}
@Override
public String getPassword() {
return user.getPassword();
}
@Override
public String getUsername() {
return user.getUsername();
}
@Override
public boolean isAccountNonExpired() {
return true;
}
@Override
public boolean isAccountNonLocked() {
return true;
}
@Override
public boolean isCredentialsNonExpired() {
return true;
}
@Override
public boolean isEnabled() {
return true;
}
}
'Spring > Spring Security' 카테고리의 다른 글
[Spring Security] Authentication 객체 타입 (0) | 2023.02.10 |
---|---|
[Spring Security] UserDetailsService (0) | 2023.02.01 |
[Spring Security] SecurityConfig (0) | 2023.01.31 |
[Spring Security] 전반적 Flow (0) | 2023.01.21 |
[Spring Security] JWT 개념 정리 (0) | 2023.01.19 |